College code
The value of pi
We all learned to write code to calculate the value of pi, a nice way to burn some cycles. But the lesson here was raw processing power or even efficient code is nothing compared to a good algorithm. The choice of equation will make the task a magnitude harder or easier.
So the story was, as an exercise, we were asked by our calculus professor to write a program that will calculate the value of pi. Whoever had the most digits at the end of the week wins.
Didn't have a C++ compiler at the time on my machine, so wrote this qbasic code shown here which survived to this day via found backups.
The actual code I used for the homework was indeed in C++ (sadly not available now) and the clever part (I thought at the time, but in fact NOT) was that I used gcc running on the University's mail server instead of my home machine, which is the approach of more hardware cycle vs. better algorithm. Lesson learned.
Those who watched TOS will remember how Spock kept the ship's misbehaving computer busy by asking it to calculate the value of pi to the last digit (which by the way, has been demostrated to be rather pointless as the application of such a high precision of pi is very limited).
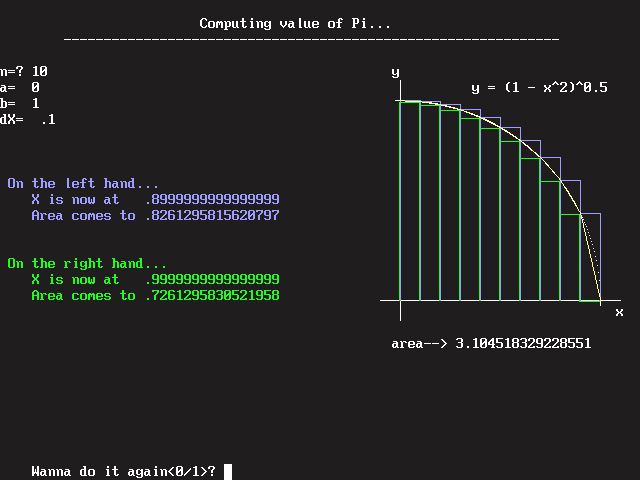
'Written by Feipang Ko February 13, 1998 All Rights Reserved (c)
'Graphics added on February 14, 1998 since I have no Valentine
'This is an attempt to use calculus and qbasic to approximate the
'value of PI
'
'
SCREEN 12
DO
PRINT
PRINT ' Computing value of Pi...'
PRINT '--------------------------------------------------------------'
PRINT
LOCATE 10, 10
INPUT 'what is the value of n'; n#
'INPUT 'what is the value of a'; a#
'INPUT 'what is the value of b'; b#
a# = 0
b# = 1
delta# = (b# - a#) / n# 'establish delta x
LOCATE 14, 13
PRINT 'delta x = '; delta#
'-------------------Axis generation section----------------------------------
'drawing the axis
'life begins at 400, 300 (backward for y, remember to 480-everything)
LINE (380, 300)-(620, 300) 'the x axis
LINE (400, 320)-(400, 80) 'the y axis
'----draws marks of delta Xs on the x axis
LINE (600, 305)-(600, 300) 'the very last mark
'FOR mark = a# TO b# STEP delta#
' xmark = (mark * 200) + 400 'at every delta Xs
' LINE (xmark, 305)-(xmark, 300)
'NEXT mark
'draws mark on y axis
LINE (395, 100)-(400, 100)
'---------------------------------------------------------------------------
x# = 0
AREA# = 0 'initialize the stuffs
FOR xpoint = 400 TO 600 STEP 1 'draw up the curve first
ypoint = 300 - (SQR(1 - ((xpoint - 400) / 200) ^ 2) * 200)
PSET (xpoint, ypoint), 15
NEXT xpoint
DO
'LEFT HAND SUM
LOCATE 15, 11
PRINT 'X is now at '; x#;
bar# = SQR(1 - x# ^ 2) * delta#
curveY = 300 - (bar# / delta#) * 200
curveX = (x# * 200) + 400
COLOR 9
LINE (curveX, curveY)-(curveX + (delta# * 200), curveY)
LINE (curveX + (delta# * 200), 300)
COLOR 15
AREA# = AREA# + bar#
LOCATE 16, 11
PRINT ' AREA NOW-->'; AREA#
x# = x# + delta#
LOOP WHILE x# < (b# - delta# / 2) 'A QUICK AND VERY DIRTY WAY
'TO SOLVE THE ROUNDING PROBLEM
'LIKE, 0.9999999 IS STILL < 1
LOCATE 20, 20
PRINT 'area-->'; AREA# * 4 '2 * pi is full area, area# is 1/2
'BEEP
INPUT 'again? <0/1>'; again
PRINT ' '
CLS
LOOP WHILE (again <> 0) 'repeat loop
Gravity simulation
Yes, BASIC. I am that old and unashamed now, that young and simple then, but when I saw the Satellite Orbit Simulator, the geek in me had to have it. When I can't find it to buy, I had to write it. A C++ compiler wasn't free back then on a Wintel PC, which is what I have besides the Tandy Color Computer III, so QBasic was what I have to use.
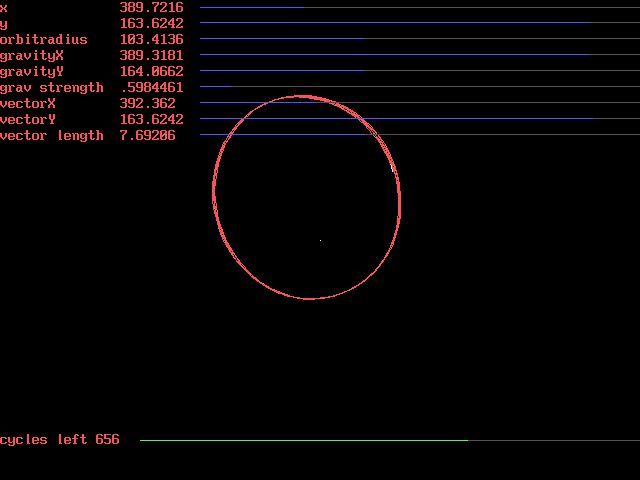
SCREEN 12
PSET (320, 240), 15
'LINE (315, 235)-(325, 235), 15 'drawing the square sun
'LINE (325, 235)-(325, 245), 15
'LINE (325, 245)-(315, 245), 15
'LINE (315, 245)-(315, 235), 15
y = 95
x = 305 'initial satellite position
lastvectorx = x + 5
lastvectory = y - 0
counter = 0
DO
PSET (x, y), 15 'paint start position
orbitradius = ((x - 320) ^ 2 + (y - 240) ^ 2) ^ .5
IF orbitradius = 0 THEN orbitradius = 1
'-------gravity---------
gravitylength = 6400 / orbitradius ^ 2
IF INKEY$ = 's' THEN
gravitylength = gravitylength / 2
ELSE IF INKEY$ = 'x' THEN gravitylength = gravitylength * 3
ELSE
gravitylength = gravitylength
END IF
gravitypointy = y - (((y - 240) * gravitylength) / orbitradius)
gravitypointx = x - (((x - 320) * gravitylength) / orbitradius)
'PSET (gravitypointx, gravitypointy), 15
'LINE (x, y)-(gravitypointx, gravitypointy), 15
'--------vector---------
'PSET (lastvectorx, lastvectory), 11
vectorx = lastvectorx + (gravitypointx - x)
vectory = lastvectory + (gravitypointy - y)
IF INKEY$ = 'a' THEN
vectorx = vectorx * 1.001
vectory = vectory * 1.001
ELSEIF INKEY$ = 'z' THEN
vectory = vectory / 2
vectorx = vectorx / 2
ELSE
vectory = vectory
vectorx = vectorx
END IF
PSET (vectorx, vectory), 13
LINE (x, y)-(vectorx, vectory), 15
vectorlength = ((vectorx - x) ^ 2 + (vectory - y) ^ 2) ^ .5
'--------trace----------
COLOR 9
LOCATE 1, 1
PRINT 'x', x
LINE (200, 22)-(640, 22), 8
LINE (200, 22)-(200 + x, 22), 9
LOCATE 2, 1
PRINT 'y', y
LINE (200, 38)-(640, 38), 8
LINE (200, 38)-(200 + y, 38), 9
LOCATE 3, 1
PRINT 'orbitradius', orbitradius
LINE (200, 7)-(640, 7), 8
LINE (200, 7)-(200 + orbitradius, 7), 9
LOCATE 4, 1
PRINT 'gravityX', gravitypointx
LINE (200, 54)-(640, 54), 8
LINE (200, 54)-(200 + gravitypointx, 54), 9
LOCATE 5, 1
PRINT 'gravityY', gravitypointy
LINE (200, 70)-(640, 70), 8
LINE (200, 70)-(200 + gravitypointy, 70), 9
LOCATE 6, 1
PRINT 'grav strength', gravitylength
LINE (200, 86)-(640, 86), 8
LINE (200, 86)-(200 + gravitylength * 50, 86), 9
LOCATE 7, 1
PRINT 'vectorX', vectorx
LINE (200, 118)-(640, 118), 8
LINE (200, 118)-(200 + vectorx, 118), 9
LOCATE 8, 1
PRINT 'vectorY', vectory
LINE (200, 134)-(640, 134), 8
LINE (200, 134)-(200 + vectory, 134), 9
LOCATE 9, 1
PRINT 'vector length', vectorlength
LINE (200, 102)-(640, 102), 8
LINE (200, 102)-(200 + vectorlength * 15, 102), 9
LOCATE 28, 1
PRINT 'cycles left'; 1000 - counter
LINE (140, 440)-(640, 440), 10
LINE (640, 440)-(640 - counter / 2, 440), 8
'------next loop-------
LINE (x, y)-(vectorx, vectory), 12
lastvectorx = vectorx * 2 - x
lastvectory = vectory * 2 - y
x = vectorx
y = vectory
'IF x > 640 THEN END
counter = counter + 1
IF counter = 1000 THEN CLS
IF counter > 1010 THEN counter = 0
IF INKEY$ = 'q' THEN END
LOOP
'0 0 Black 0(c) Off
'1 1 Blue Underlined(d)
'2 2 Green 1(c) On(d)
'3 3 Cyan 1(c) On(d)
'4 4 Red 1(c) On(d)
'5 5 Magenta 1(c) On(d)
'6 6 Brown 1(c) On(d)
'7 7 White 1(c) On(d)
'8 8 Gray 0(c) Off
'9 9 Light Blue High-intensity
'10 10 Light green 2(c) High-intensity
'11 11 Light cyan 2(c) High-intensity
'12 12 Light red 2(c) High-intensity
'13 13 Light magenta 2(c) High-intensity
'14 14 Yellow 2(c) High-intensity
'15 15 High-intensity 0(c) Off
' white








Views: 537
Replies coming soon